How to use DynamicConfig classes in the catalogue
This how-to guide will introduce you to DynamicConfig classes in the catalogue. In the following explanations, we assume that you’re already familiar with the knowledge from the basic usage tutorial. So work through this first if you haven’t already done so.
The complete source code for this tutorial is available here.
Creating folders
In a first step, the folders are created. The final folder structure will look like this.
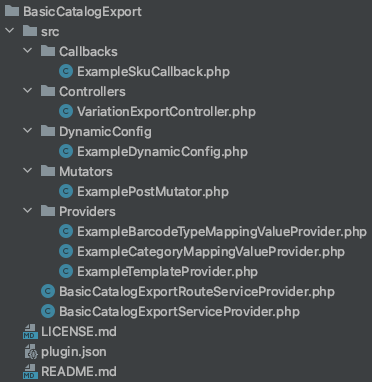
What is a DynamicConfig class?
A DynamicConfig class should be used to provide necessary logic for the export, which cannot be defined in the template scope. This can have multiple reasons. They might depend on the specifics of a given catalogue or on other settings inside the system. The need for the specific location for this logic comes from the fact that the catalogue itself can trigger exports (e.g. through the UI). This means that any logic outside the catalogue’s scope would not be applicable in exports that are triggered directly by the catalogue.
This would cause different export results depending on the trigger which will lead to more problems down the line. Thus, the DynamicConfig provides a spot to inject your logic directly before the export process is triggered.
If you have any additional logic outside of the DynamicConfig class, make sure that the isPreviewable()
method of your template provider returns false, since this blocks all export triggers in the specific catalogue scope.
Using DynamicConfig in your template
To implement DynamicConfig in your template, just overwrite the getDynamicConfig()
method in your template provider and create an instance of your own class that implements the CatalogDynamicConfigContract.
In this class you need to create two methods, applyDynamicConfig()
and applyDynamicPreviewConfig()
. These methods receive identical parameters: the catalog, the template and an instance of CatalogExportService.
With these you can add additional filters to the template, or allow or forbid specific keys in the export service. In case you need a logic which is not maintainable on a template level before the export gets triggered, put the logic into the DynamicConfig.
The difference between the two methods is that applyDynamicConfig()
is called in a general export, while applyDynamicPreviewConfig()
is only called if a user requests a preview. If you do not need specific logic in a preview you can just call applyDynamicConfig()
from there.
As an example, we will reduce the exported keys to stock and sku, which could be very helpful in case we want to write a stock export. The class could look like this:
<?php
namespace BasicCatalogExport\DynamicConfig;
use Plenty\Modules\Catalog\Contracts\CatalogDynamicConfigContract;
use Plenty\Modules\Catalog\Contracts\CatalogExportServiceContract;
use Plenty\Modules\Catalog\Contracts\TemplateContract;
use Plenty\Modules\Catalog\Models\Catalog;
/**
* Class ExampleDynamicConfig
* @package BasicCatalogExport\DynamicConfig
*/
class ExampleDynamicConfig implements CatalogDynamicConfigContract
{
/**
* @param TemplateContract $template
* @param Catalog $catalog
* @param CatalogExportServiceContract $catalogExportService
*/
public function applyDynamicConfig(
TemplateContract $template,
Catalog $catalog,
CatalogExportServiceContract $catalogExportService
) {
// We could add new filters
//$filterContainer = $template->getFilterContainer();
//$filterContainer->addFilterBuilder();
// In a real scenario we will probably have some conditions here
// This will remove all keys from the export that are not specifically stated in here
$catalogExportService->allowExportKeys('sku', 'stock');
}
/**
* @param TemplateContract $template
* @param Catalog $catalog
* @param CatalogExportServiceContract $catalogExportService
*/
public function applyDynamicPreviewConfig(
TemplateContract $template,
Catalog $catalog,
CatalogExportServiceContract $catalogExportService
) {
$this->applyDynamicConfig($template, $catalog, $catalogExportService);
}
}
As mentioned above, we now also need to overwrite the getDynamicConfig()
method in our template provider. This method will look like this:
public function getDynamicConfig(): CatalogDynamicConfigContract
{
return pluginApp(ExampleDynamicConfig::class);
}
If we now create a catalogue and fill some fields with sources, we will see in the preview that we still only receive stock and sku.
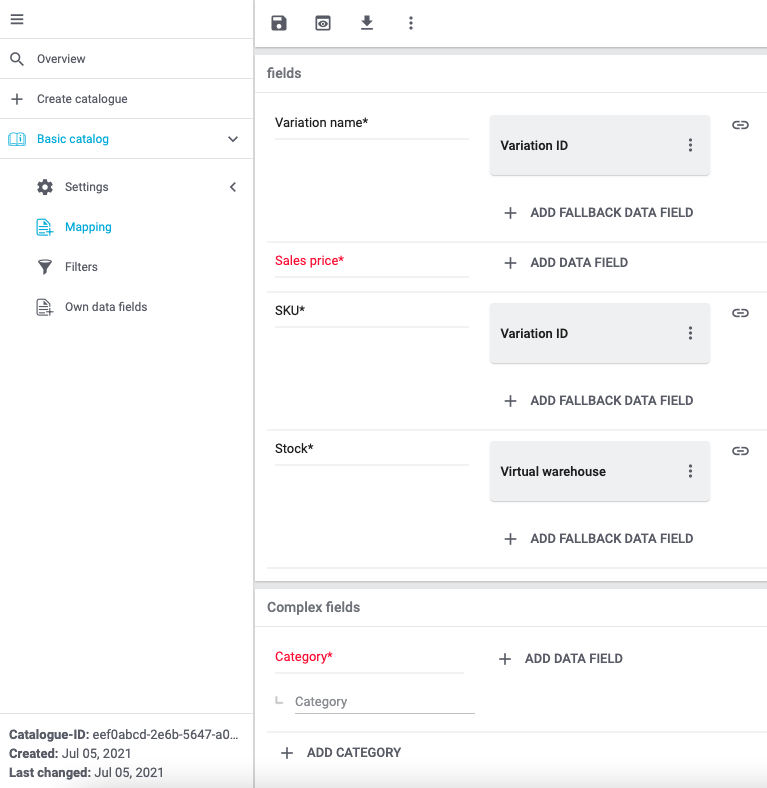
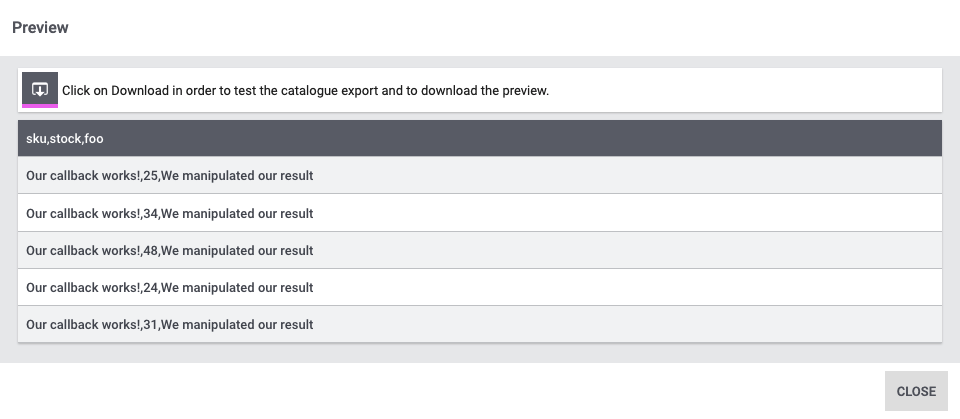
As you can see, the behaviour of our data manipulation will not be influenced by the restriction of specific keys.
Providing your export configuration
You are now able to provide your conditional export configuration in your template. This enables your users to benefit from all the possible catalogue export triggers.
Further reading
Another topic that might be interesting for you at this point are ResultConverters, which you can use to manipulate your result’s file type and change it for example to CSV.